概要
このブログ記事では、PythonのTkinterライブラリを使ったGUIプログラミングの基本を学びます。Tkinterの主要なウィジェットの使い方と、プログラムを作成する際のポイントについて解説します。
1. Tkinterとは?
Tkinterの概要
Tkinterは、Pythonに標準で付属しているGUIライブラリです。GUIとは、グラフィカル・ユーザー・インターフェースの略で、ボタンやテキストボックスなどのグラフィカルな要素を使って、ユーザーとコンピュータがやり取りする仕組みです。
Tkinterを使う理由
Tkinterは簡単に使えるため、プログラミング初心者でもすぐに学べます。また、Pythonに標準で付属しているため、追加のインストールが不要です。
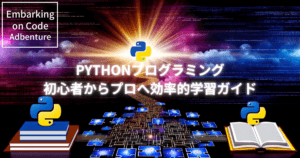
2. Tkinterの基本的な使い方
Tkinterのインストールとセットアップ
TkinterはPythonに標準で付属しているため、追加のインストールは必要ありません。
最初のウィンドウを作成する
以下のコードを実行してみましょう。
import tkinter as tk
# ウィンドウを作成
root = tk.Tk()
root.title("Hello, Tkinter!")
# ウィンドウを表示
root.mainloop()
解説
import tkinter as tk
でTkinterモジュールをインポートします。root = tk.Tk()
でウィンドウを作成します。root.title("Hello, Tkinter!")
でウィンドウのタイトルを設定します。root.mainloop()
でウィンドウを表示し、ユーザーの操作を待ちます。
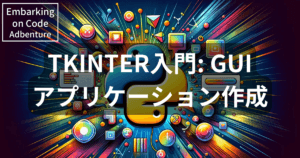
3. 主要なTkinterウィジェット
Label
Labelウィジェットは、テキストを表示するためのウィジェットです。
import tkinter as tk
root = tk.Tk()
root.title("Label Example")
# Labelウィジェットを作成
label = tk.Label(root, text="Hello, Tkinter!")
label.pack()
root.mainloop()
解説
label = tk.Label(root, text="Hello, Tkinter!")
でLabelウィジェットを作成します。text
オプションで表示するテキストを指定します。label.pack()
でLabelウィジェットをウィンドウに配置します。
Button
Buttonウィジェットは、クリックできるボタンを作成します。
import tkinter as tk
root = tk.Tk()
root.title("Button Example")
def on_button_click():
print("Button clicked!")
# Buttonウィジェットを作成
button = tk.Button(root, text="Click Me!", command=on_button_click)
button.pack()
root.mainloop()
解説
def on_button_click():
でボタンがクリックされたときの処理を定義します。button = tk.Button(root, text="Click Me!", command=on_button_click)
でButtonウィジェットを作成します。command
オプションでクリック時の処理を指定します。button.pack()
でButtonウィジェットをウィンドウに配置します。
Entry
Entryウィジェットは、一行のテキスト入力フィールドを作成します。
import tkinter as tk
root = tk.Tk()
root.title("Entry Example")
# Entryウィジェットを作成
entry = tk.Entry(root)
entry.pack()
root.mainloop()
解説
entry = tk.Entry(root)
でEntryウィジェットを作成します。entry.pack()
でEntryウィジェットをウィンドウに配置します。
ファイルダイアログ
ファイルダイアログは、ファイルを開いたり保存したりするためのウィジェットです。tkinter.filedialog
モジュールを使用します。
ファイルを開くダイアログ
import tkinter as tk
from tkinter import filedialog
def open_file():
file_path = filedialog.askopenfilename()
if file_path:
with open(file_path, 'r') as file:
print(file.read())
root = tk.Tk()
root.title("File Dialog Example")
open_button = tk.Button(root, text="Open File", command=open_file)
open_button.pack()
root.mainloop()
解説
from tkinter import filedialog
でfiledialogモジュールをインポートします。def open_file():
でファイルを開く処理を定義します。filedialog.askopenfilename()
でファイルダイアログを開きます。open_button = tk.Button(root, text="Open File", command=open_file)
でButtonウィジェットを作成し、ファイルを開く処理を指定します。open_button.pack()
でButtonウィジェットをウィンドウに配置します。
ファイルを保存するダイアログ
import tkinter as tk
from tkinter import filedialog
def save_file():
file_path = filedialog.asksaveasfilename(defaultextension=".txt")
if file_path:
with open(file_path, 'w') as file:
file.write("Hello, Tkinter!")
root = tk.Tk()
root.title("File Dialog Example")
save_button = tk.Button(root, text="Save File", command=save_file)
save_button.pack()
root.mainloop()
解説
def save_file():
でファイルを保存する処理を定義します。filedialog.asksaveasfilename()
でファイル保存ダイアログを開きます。save_button = tk.Button(root, text="Save File", command=save_file)
でButtonウィジェットを作成し、ファイルを保存する処理を指定します。save_button.pack()
でButtonウィジェットをウィンドウに配置します。
4. ウィジェットの配置方法
pack
packメソッドはウィジェットをウィンドウに簡単に配置するための方法です。
import tkinter as tk
root = tk.Tk()
root.title("Pack Example")
label1 = tk.Label(root, text="Label 1")
label1.pack()
label2 = tk.Label(root, text="Label 2")
label2.pack()
root.mainloop()
解説
label1.pack()
とlabel2.pack()
で、Labelウィジェットをウィンドウに順番に配置します。
grid
gridメソッドはウィジェットをグリッド状に配置します。
import tkinter as tk
root = tk.Tk()
root.title("Grid Example")
label1 = tk.Label(root, text="Label 1")
label1.grid(row=0, column=0)
label2 = tk.Label(root, text="Label 2")
label2.grid(row=1, column=1)
root.mainloop()
解説
label1.grid(row=0, column=0)
でLabelウィジェットをグリッドの1行1列目に配置します。label2.grid(row=1, column=1)
でLabelウィジェットをグリッドの2行2列目に配置します。
place
placeメソッドはウィジェットを絶対位置に配置します。
import tkinter as tk
root = tk.Tk()
root.title("Place Example")
label1 = tk.Label(root, text="Label 1")
label1.place(x=50, y=50)
label2 = tk.Label(root, text="Label 2")
label2.place(x=100, y=100)
root.mainloop()
解説
label1.place(x=50, y=50)
でLabelウィジェットをウィンドウ内の(50, 50)の位置に配置します。label2.place(x=100, y=100)
でLabelウィジェットをウィンドウ内の(100, 100)の位置に配置します。
5. イベントハンドリング
ボタンのクリックイベント
import tkinter as tk
root = tk.Tk()
root.title("Button Click Example")
def on_button_click():
print("Button clicked!")
button = tk.Button(root, text="Click Me!", command=on_button_click)
button.pack()
root.mainloop()
解説
def on_button_click():
でボタンがクリックされたときの処理を定義します。button = tk.Button(root, text="Click Me!", command=on_button_click)
でButtonウィジェットを作成し、クリック時の処理を指定します。button.pack()
でButtonウィジェットをウィンドウに配置します。
キーボードイベント
import tkinter as tk
root = tk.Tk()
root.title("Keyboard Event Example")
def on_key_press(event):
print(f"Key pressed: {event.keysym}")
root.bind("<KeyPress>", on_key_press)
root.mainloop()
解説
def on_key_press(event):
でキーが押されたときの処理を定義します。event.keysym
で押されたキーを取得します。root.bind("<KeyPress>", on_key_press)
でキーが押されたときにon_key_press
関数を呼び出すようにします。
マウスイベント
import tkinter as tk
root = tk.Tk()
root.title("Mouse Event Example")
def on_mouse_click(event):
print(f"Mouse clicked at: ({event.x}, {event.y})")
root.bind("<Button-1>", on_mouse_click)
root.mainloop()
解説
def on_mouse_click(event):
でマウスがクリックされたときの処理を定義します。event.x
とevent.y
でクリックされた位置を取得します。root.bind("<Button-1>", on_mouse_click)
でマウスの左ボタンがクリックされたときにon_mouse_click
関数を呼び出すようにします。
6. ウィジェットのスタイリング
ウィジェットのカスタマイズ
import tkinter as tk
root = tk.Tk()
root.title("Styling Example")
label = tk.Label(root, text="Styled Label", font=("Arial", 24), fg="blue")
label.pack()
root.mainloop()
解説
label = tk.Label(root, text="Styled Label", font=("Arial", 24), fg="blue")
でLabelウィジェットを作成し、フォントと文字色を設定します。label.pack()
でLabelウィジェットをウィンドウに配置します。
テーマとスタイルの適用
import tkinter as tk
from tkinter import ttk
root = tk.Tk()
root.title("Theme Example")
style = ttk.Style()
style.theme_use('clam')
button = ttk.Button(root, text="Styled Button")
button.pack()
root.mainloop()
解説
from tkinter import ttk
でttkモジュールをインポートします。style = ttk.Style()
でスタイルオブジェクトを作成し、style.theme_use('clam')
でテーマを設定します。button = ttk.Button(root, text="Styled Button")
でttk.Buttonウィジェットを作成します。button.pack()
でButtonウィジェットをウィンドウに配置します。
Tkinterでは、ttkモジュールを使用してテーマとスタイルを設定することができます。ttkは、Tkinterのテーマ対応ウィジェットを提供するサブモジュールです。ここでは、いくつかの一般的なテーマとスタイルについて説明します。
1. テーマ (Themes)
テーマは、ウィジェットの全体的な見た目を変更するために使用されます。以下に、Tkinterで使用できるいくつかのテーマを紹介します。
- default:
- Tkinterのデフォルトテーマです。シンプルでクラシックな見た目を持ちます。
- clam:
- クリーンでモダンな見た目のテーマです。控えめなデザインで、多くのアプリケーションで使いやすいです。
- alt:
- テーマのバリエーションの一つで、ボタンやエントリーフィールドのスタイルが微妙に異なります。
- classic:
- 古典的なTkinterのテーマです。シンプルでレトロな見た目が特徴です。
- vista:
- Windows Vistaに似たテーマです。Windowsユーザーには親しみやすいデザインです。
- xpnative:
- Windows XPに似たテーマです。Windows XPのスタイルに似た外観を持っています。
2. スタイル (Styles)
スタイルは、特定のウィジェットの見た目を詳細にカスタマイズするために使用されます。ttkのスタイルシステムを使うと、フォント、色、パディングなどを設定できます。
以下に、スタイルの基本的な設定例を紹介します。
import tkinter as tk
from tkinter import ttk
root = tk.Tk()
root.title("Theme and Style Example")
# テーマを設定
style = ttk.Style()
style.theme_use('clam')
# ボタンスタイルをカスタマイズ
style.configure('TButton', font=('Helvetica', 12), foreground='blue')
# ボタンを作成
button = ttk.Button(root, text="Styled Button")
button.pack(pady=20)
root.mainloop()
スタイル設定のオプション
- font: フォントの種類、サイズ、スタイル(例:
('Helvetica', 12, 'bold')
)。 - foreground: テキストの色(例:
'blue'
)。 - background: 背景色(例:
'white'
)。 - padding: ウィジェット内の余白(例:
10
または(10, 5)
)。 - relief: ウィジェットの境界線スタイル(例:
'flat'
,'raised'
,'sunken'
,'solid'
)。
テーマとスタイルの使い分け
テーマはアプリケーション全体の統一感を出すために使用し、スタイルは特定のウィジェットを目立たせたり、特定の機能を強調するために使用します。テーマを選んだ後、必要に応じてスタイルをカスタマイズすることで、見た目が整った使いやすいインターフェースを作成できます。
Tkinterのテーマとスタイルをうまく組み合わせて、魅力的で使いやすいGUIアプリケーションを作成してみてください。
7. ファイルの読み込みと保存
ファイルを開くダイアログ
import tkinter as tk
from tkinter import filedialog
def open_file():
file_path = filedialog.askopenfilename()
if file_path:
with open(file_path, 'r') as file:
print(file.read())
root = tk.Tk()
root.title("Open File Example")
open_button = tk.Button(root, text="Open File", command=open_file)
open_button.pack()
root.mainloop()
解説
from tkinter import filedialog
でfiledialogモジュールをインポートします。def open_file():
でファイルを開く処理を定義します。filedialog.askopenfilename()
でファイルダイアログを開きます。open_button = tk.Button(root, text="Open File", command=open_file)
でButtonウィジェットを作成し、ファイルを開く処理を指定します。open_button.pack()
でButtonウィジェットをウィンドウに配置します。
ファイルを保存するダイアログ
import tkinter as tk
from tkinter import filedialog
def save_file():
file_path = filedialog.asksaveasfilename(defaultextension=".txt")
if file_path:
with open(file_path, 'w') as file:
file.write("Hello, Tkinter!")
root = tk.Tk()
root.title("Save File Example")
save_button = tk.Button(root, text="Save File", command=save_file)
save_button.pack()
root.mainloop()
解説
def save_file():
でファイルを保存する処理を定義します。filedialog.asksaveasfilename()
でファイル保存ダイアログを開きます。save_button = tk.Button(root, text="Save File", command=save_file)
でButtonウィジェットを作成し、ファイルを保存する処理を指定します。save_button.pack()
でButtonウィジェットをウィンドウに配置します。
8. 実践的なサンプルプログラム
簡単なToDoリストアプリの作成
import tkinter as tk
root = tk.Tk()
root.title("ToDo List")
tasks = []
def add_task():
task = entry.get()
if task != "":
tasks.append(task)
listbox.insert(tk.END, task)
entry.delete(0, tk.END)
def delete_task():
selected_task_index = listbox.curselection()
if selected_task_index:
tasks.pop(selected_task_index[0])
listbox.delete(selected_task_index)
entry = tk.Entry(root)
entry.pack()
add_button = tk.Button(root, text="Add Task", command=add_task)
add_button.pack()
delete_button = tk.Button(root, text="Delete Task", command=delete_task)
delete_button.pack()
listbox = tk.Listbox(root)
listbox.pack()
root.mainloop()
解説
tasks = []
でタスクのリストを作成します。def add_task():
でタスクを追加する処理を定義します。entry.get()
でテキスト入力フィールドからタスクを取得し、リストに追加します。def delete_task():
でタスクを削除する処理を定義します。選択されたタスクをリストから削除します。entry = tk.Entry(root)
でEntryウィジェットを作成し、entry.pack()
で配置します。add_button = tk.Button(root, text="Add Task", command=add_task)
でタスク追加ボタンを作成し、add_button.pack()
で配置します。delete_button = tk.Button(root, text="Delete Task", command=delete_task)
でタスク削除ボタンを作成し、delete_button.pack()
で配置します。listbox = tk.Listbox(root)
でListboxウィジェットを作成し、listbox.pack()
で配置します。
電卓アプリの作成
import tkinter as tk
root = tk.Tk()
root.title("Calculator")
expression = ""
def add_to_expression(symbol):
global expression
expression += str(symbol)
equation.set(expression)
def evaluate_expression():
global expression
try:
result = str(eval(expression))
equation.set(result)
expression = result
except:
equation.set("error")
expression = ""
def clear_expression():
global expression
expression = ""
equation.set("")
equation = tk.StringVar()
entry = tk.Entry(root, textvariable=equation)
entry.grid(row=0, column=0, columnspan=4)
buttons = [
'7', '8', '9', '/',
'4', '5', '6', '*',
'1', '2', '3', '-',
'0', '.', '=', '+'
]
row = 1
col = 0
for button in buttons:
action = lambda x=button: add_to_expression(x) if x != "=" else evaluate_expression()
tk.Button(root, text=button, command=action).grid(row=row, column=col, sticky="nsew")
col += 1
if col > 3:
col = 0
row += 1
tk.Button(root, text="C", command=clear_expression).grid(row=row, column=col, columnspan=4, sticky="nsew")
root.mainloop()
解説
expression = ""
で計算式を保存する変数を初期化します。def add_to_expression(symbol):
で計算式に記号を追加する処理を定義します。expression
に記号を追加し、equation.set(expression)
でエントリーフィールドに表示します。def evaluate_expression():
で計算式を評価する処理を定義します。eval(expression)
を使って計算し、結果を表示します。def clear_expression():
で計算式をクリアする処理を定義します。equation = tk.StringVar()
で文字列変数を作成し、計算式を保存します。entry = tk.Entry(root, textvariable=equation)
でEntryウィジェットを作成し、計算式を表示します。buttons
リストに電卓のボタンを定義します。- ボタンをグリッドに配置し、クリック時の処理を指定します。
9. トラブルシューティングとよくある質問
よくあるエラーとその対処法
- エラー1:
No module named 'tkinter'
- 解決法:
pip install tk
を試してみてください。
- 解決法:
- エラー2: ウィジェットが表示されない
- 解決法:
pack
,grid
,place
メソッドを正しく使っているか確認してください。
- 解決法:
参考資料とリソース
10. まとめと次のステップ
この記事では、PythonのTkinterウィジェットの基本的な使い方について学びました。次のステップとして、より複雑なアプリケーションを作成したり、他のGUIライブラリを学んだりすることをおすすめします。
プログラミングに興味があるけれど、何から始めればいいかわからない方に最適な一冊が「スッキリわかるPython入門 第2版」です。以下のポイントを参考にしてください。
本書の特徴とメリット
- シリーズ累計90万部突破
多くの読者に支持され、信頼されている大人気入門書の改訂版。 - 初心者でもわかりやすい解説
基本的な「コツ」を丁寧に説明し、迷わず学習を進められます。 - 実践的な「しくみ」の理解
プログラミングの基礎だけでなく、実際の開発に役立つ知識を習得可能。 - 「落とし穴」の回避
初心者が陥りがちな間違いをカバーし、安心して学習を進められる内容。
実際の読者の声
- 現役プログラミング教室の先生も推薦!
「この本を読んでPCスキルをマスターすれば、それでメシを食えますよ」という評価もあるほどの内容。面白くて勉強になるとの声が多い。
プログラミング教育において、多くの初学者が挫折する理由をご存じでしょうか?実は、それには多くの共通点があります。テックジムは、その問題点を深く理解し、20年以上にわたって蓄積してきた経験をもとに、誰もが安心して学べるプログラミング講座を提供しています。
テックジムは、ただの学習場ではありません。プログラミングを始めたい方や、より高いレベルに達したい方々に向けた、実践的な学びの場です。私たちが提供するカリキュラムは、初心者が直面する課題や躓きやすいポイントを徹底的に研究し、それを解決するためにデザインされています。
多くのプログラミングスクールが、フレームワークや複雑な技術から始めることで、学習者に過度な負担をかけ、結果として挫折を生む原因となっています。テックジムでは、まずは本当に重要な基礎からスタートすることで、無理なくスキルを積み上げていくことができます。例えば、関数やクラスといったプログラミングの核心部分をしっかりと理解し、それを使いこなすための時間を十分に確保しています。
これにより、受講生たちは無駄な混乱を避け、確実にスキルを身につけていくことができるのです。テックジムでの学びは、単なる知識の詰め込みではなく、実際に「できる」ことを目指した実践的なトレーニングです。
テックジムのPythonプログラミング講座は、経験と実績が詰まった講座です。初心者でも安心して参加でき、確実にステップアップできるこの講座で、あなたもプログラミングの世界に飛び込んでみませんか?
プログラミング学習に挑戦した多くの人が、途中で挫折してしまうことがあります。これは、難解なフレームワークや複雑な概念にいきなり取り組むことが主な原因です。しかし、テックジムではそのような挫折を未然に防ぐため、独自のカリキュラムを採用しています。
テックジムのカリキュラムは、まず基礎をしっかりと固めることから始めます。関数やクラスといったプログラミングの根幹をじっくり学ぶことで、無駄な負荷をかけずに確実にスキルを身につけることができます。このアプローチにより、学習者は「何をやっているのかわからない」という混乱を避け、自信を持って次のステップに進むことができます。
また、テックジムでは、段階的にスキルを積み上げることで、学習の進行に伴う負担を最小限に抑えています。その結果、無理なく、着実にプログラミングの世界で成功を収めることができるのです。
テックジムのプログラミング講座は、学ぶことの楽しさを実感しながら、挫折せずに成長できる最適な環境を提供します。
プログラミング学習において、最新技術の活用は欠かせません。テックジムでは、ChatGPTを用いた学習サポートを取り入れています。ChatGPTは、あらゆる質問に即座に答え、コードのバグ解決もスムーズにサポートします。これにより、効率的に学習を進めることが可能です。
しかし、テックジムの強みは、これだけではありません。どんなに優れたAIでも、人間のコーチによる個別サポートの価値は計り知れません。テックジムでは、経験豊富なプロのコーチがあなたの学習を支えます。プログラミングの基礎から応用まで、丁寧な指導と的確なフィードバックを提供し、あなたが抱える疑問や課題を一つ一つ解決していきます。
このように、最新の技術とプロのコーチングを組み合わせることで、テックジムでは、効率的でありながらも確実にスキルを身につけることができる学習環境を提供しています。
テックジムで学びながら、最先端のAI技術とプロの指導のベストな融合を体験してみませんか?
テックジムのPythonプログラミング講座は、その効果と実績で多くの受講生から高い評価を受けています。8月には180名を超える方々がこの講座にエントリーし、その人気と信頼の高さを証明しています。
この講座では、受講生が着実にスキルを身につけ、成長していることを実感できるカリキュラムを提供しています。プログラミングの基礎から実践的な応用まで、段階的に学べる内容は、初心者から経験者まで幅広く対応しています。また、学んだ知識をすぐに実践に移せる環境を整えており、学習の成果をリアルタイムで確認できるのも大きな特徴です。
テックジムの講座を受講した多くの方々が、「理解が深まった」「自信を持ってコードを書けるようになった」といった喜びの声を寄せています。これまでに培った経験と実績を活かし、受講生一人ひとりが成功への第一歩を踏み出せるよう全力でサポートしています。
あなたも、この成果を実感できるカリキュラムで、プログラミングスキルを確実に伸ばしてみませんか?
プログラミングに興味はあるけれど、いきなり本格的な学習に踏み出すのは少し不安…そんな方に最適なのが、テックジムの無料体験です。まずは気軽に始めてみたい、という方のために、テックジムではデモレッスンを提供しています。
この無料体験では、実際のカリキュラムの一部を体験し、学習の進め方や講師のサポートを実感することができます。受講前に「自分に合っているかどうか」を確認できるので、安心してスタートを切ることができます。
プログラミングが全く初めての方も、すでにある程度の経験を持っている方も、まずはこの無料体験で、テックジムの学びを体感してみませんか?今すぐ始める一歩が、あなたの未来を大きく変えるかもしれません。
無料体験は随時開催中です。ぜひこの機会に、新たなスキルを手に入れるための第一歩を踏み出してみてください!